Table of Contents
Introduction
Sometimes we would need to add a few more dynamic parameters to such HTTP API calls before they’re passed onto the remote API services.
- Or read something from the response before it is forwarded to the calling services.
- Or adding an Authentication header
- Or adding a timestamp query parameter at the end of all requests
- Or capturing an ETag from the response headers and preserve it
- Or reading the response headers for any other required values and so on
In such cases, it’s not a good practice to replicate the same logic in all the methods of the service.
Instead, we can encapsulate this functionality inside a utility and attach this so as to monitor all outgoing requests or incoming responses and do something.
These components are called Interceptors.
What is a HttpInterceptor?
HttpInterceptor helps intercept and handle an HttpRequest or HttpResponse. It is available in @angular/common/http library.
How to implement a HttpInterceptor in Angular with an Example
Let’s say we want to pass in a custom Header called X-Request-ID in all our Post API calls before pushing it to the network.
Custom class implementing HttpInterceptor
To do this, we create our own custom Interceptor class which implements HttpInterceptor and encapsulates this functionality.
import {
HttpInterceptor,
HttpRequest,
HttpHandler,
HttpEvent
} from '@angular/common/http';
import { Observable } from 'rxjs';
import { Guid } from 'guid-typescript';
export class PostsInterceptor implements HttpInterceptor {
intercept(req: HttpRequest<any>, next: HttpHandler):
Observable<HttpEvent<any>> {
// functionality specific to
// this Interceptor - adding a request header
if (requestPath.indexOf('posts') > -1) {
var xRequestId = Guid.create().toString();
var headers = req.headers.append("X-Request-ID", xRequestId);
req = req.clone({
headers: headers
});
}
// passes on the request to next handler
// once all the handlers are executed
// the request is now pushed into the network
// for handling by the API
return next.handle(req);
}
}
Observe that in this, we add our intermediate logic to be performed on the outgoing request.
In this case, adding a request header and then we pass on the request to be handled by the next handler.
In an Angular application, a request passes over several such in-built handlers before finally passed onto the API.
Registering Interceptor in AppModule
Finally we add this interceptor implementation inside our Module class under Providers.
@NgModule({
declarations: [
PostItemComponent,
PostListComponent,
NewPostComponent
],
imports: [
CommonModule,
RouterModule.forChild(routes),
FormsModule,
HttpClientModule
],
providers: [
{
provide: HTTP_INTERCEPTORS,
useClass: PostsInterceptor,
multi: true
}
]
})
export class PostsModule { }
When we run this and check our outgoing requests, we can see the xRequestId header added to our final Request.
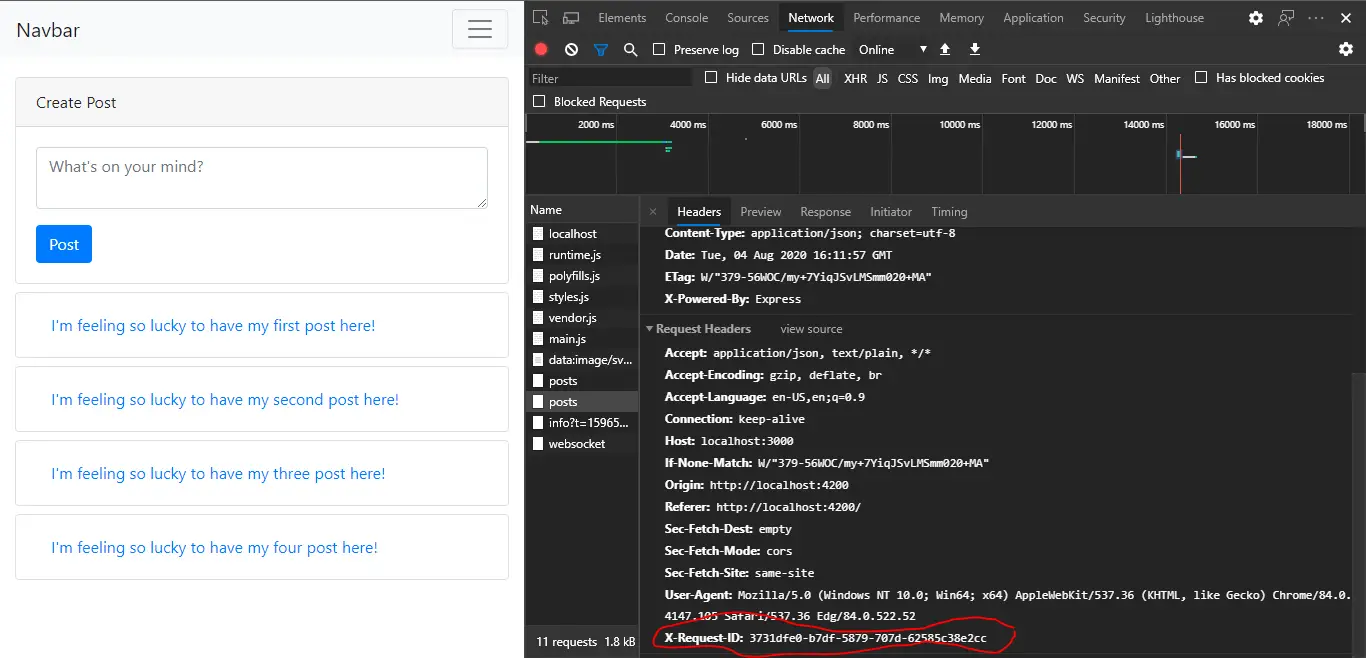
Conclusion
Apart from handling requests or responses, Interceptors can also be used to handle Errors globally before being caught at the calling components.
In this way, we can make API requests from our Angular application using the HttpClient library and add listeners for preprocessing the requests or post processing the responses using HttpInterceptors.
The code snippets used above is a part of the OpenSocialApp repository and available in GitHub – https://github.com/referbruv/opensocialapp-angular-example
Extensive features, strong community support, comprehensive documentation, and vast ecosystem of libraries and tools make Angular a valuable skill to acquire for career growth in 2023.
Check out this most popular Angular course in Udemy at a HUGE DISCOUNT for a LIMITED PERIOD – Angular – The Complete Guide (2023 Edition) exclusive for our readers!