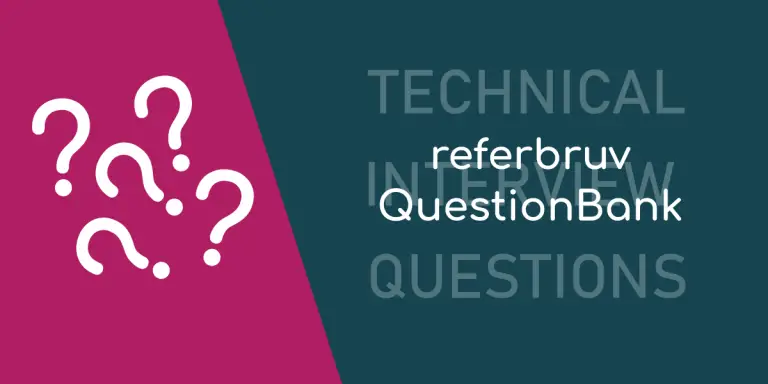
How do you detect browser in JavaScript / jQuery?
In JavaScript, we can get the browser information by means of the window.navigator object.
#properties#
window.navigator.appName
window.navigator.appCodeName
window.navigator.platform
In JavaScript, we can get the browser information by means of the window.navigator object.
#properties#
window.navigator.appName
window.navigator.appCodeName
window.navigator.platform
We can make the code unreadable to some extent by means of minification and uglify, but since angular works completely on the client end, we can't completely protect the code from being exposed.
For such cases, we can simply create a Shared service which holds the value, which one component updates and other component binds in its view.
@Injectable()
export class MyService {
myVal: string;
}
@Component()
export class Component1 {
constructor(private myService: MyService) {}
onUpdated(newVal) {
this.myService.myVal = newVal;
}
}
@Component() {
export class Component2 implements OnInit {
myVal: string;
constructor(private myService: MyService) {}
ngOnInit() {
this.myVal = this.myService.myVal;
}
}
In Angular, components can communicate with each other by any of the following means:
Reactive Forms provide a Model based approach to handle form inputs and offer an immutable approach for handling the form state at any given point of time. This facilitates having consistent and predictable form state data for easy testing.
Off late, Single-Page Applications or SPAs have become the most sought after client facing application stacks, for their light-weight and high performance nature. In this architecture, the server focuses on data logic and supplies data to the client in the form of RESTful APIs, while the client application renders the fetched data onto a fluid and dynamic layout.