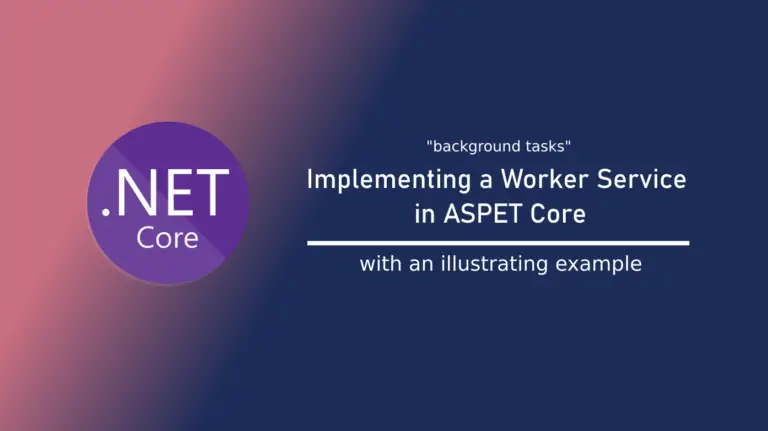
How to create a Worker Service in ASP.NET Core
In this article, let's learn about what is a Hosted Service and how we can create and use a Hosted Service inside ASP.NET Core with an example.
In this article, let's learn about what is a Hosted Service and how we can create and use a Hosted Service inside ASP.NET Core with an example.
Filters are components built into the ASP.NET Core which can help us in controlling the execution of a request at specific stages of the request pipeline. These come into picture post the middleware execution, when the MVC middleware matches a Route and a specific Action is invoked.
While the terminology might seem alien to us, but at its core its just a web service which returns data from its data source in a predefined XML schema called as RSS. In this article, let's build a simple endpoint in our ASP.NET Core WebAPI which can return RSS feed from a Posts database.
In this article, let's discuss the drawbacks with the conventional MVC routing and what Endpoint Routing brings to the table in ASP.NET Core 3.1 onwards.
We can make use of the built-in UseExceptionHandler() middleware for catching Global Errors in ASP.NET Core.
app.UseExceptionHandler(err =>
{
err.Run(async ctx =>
{
context.Response.StatusCode = 500;
context.Response.ContentType = "application/json";
// gets error detail
var ex = context.Features.Get<IExceptionHandlerPathFeature>();
var error = new MyResponseModel() { Content = ex.Error.Message };
await context.Response.WriteAsync(JsonConvert.SerializeObject(error));
});
});
Let's see how we can create and deploy our ASP.NET Core microservices in AWS using a Serverless Model via CloudFormation.
In this how-to guide, learn about how we can deploy our ASP.NET Core Web API as an AWS Lambda function and the things we need to look into for it.
In this hands-on article, let's understand how to connect and perform CRU with DynamoDB using ASP.NET Core with an illustrating example.