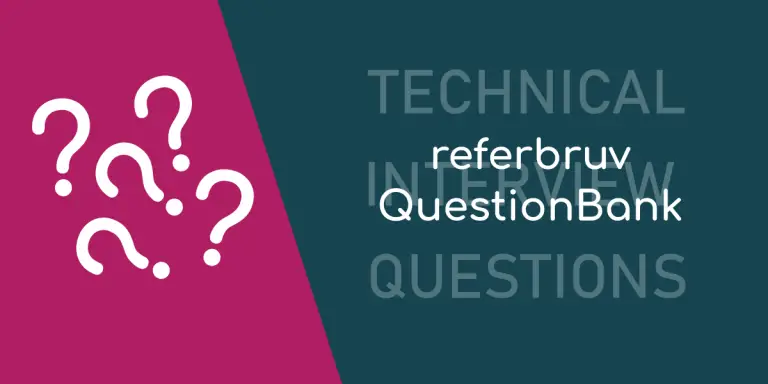
Can you unit test a private method? How do you do it?
The answer is No. We cannot unit test a private method.
- Unit Tests are designed to test the functionalities of components which are exposed to other components for consumption.
- Private methods are designed to hide functionality from the other components as a part of data abstraction.
- So its not a good practice to try unit testing a private method.
- We can instead try unit testing a public method which calls this private method and assert the overall expectation.