In Java, access specifiers are the keywords which are used to define the access scope of the method, class, or a variable within a component. With these access specifiers we can enforce security and encapsulate data or functionality from unwanted access outside of the program.
Advantages of Using Access Specifiers
The following are 4 major advantages of using access specifiers in Java –
- Information Hiding – Using access specifiers, we can hide data or information from unwanted access at a variable, method or class level. This creates a clear separation between the internals of a component (functionality or data that is internal to the class) and the exposures (the functionality that the class intentionally exposes to other classes or components)
- Access Control – As mentioned above, using access specifiers can help in creating a controlled access to the functionalities and data of a class, there by ensuring that other classes can only see what is allowed to be seen by the class.
- Inheritance – In Object Oriented Programming languages like Java, Inheritance is natively supported using which a class can extend behavior and data from another class. But sometimes we may not be interested to let any inheriting class access all the information from the class that it is inheriting. Using access specifiers we can control what any class can extend and what it still can’t access.
- Package Access – Sometimes you may want to have certain fields be accessed by other classes but only within the package or assembly that the class belongs to. This ensures that the information is not readily available to other packages which are not a part of the feature being shipped.
What are the different types of access specifiers in Java?
Technically, there are four access specifiers in java.
Public
The classes, methods, or variables which are defined as public, can be accessed by any class or method.
Protected
Protected can be accessed by the class of the same package, or by the sub-class of this class, or within the same class.
Default
Default are accessible within the package only. By default, all the classes, methods, and variables are of default scope.
Private
The private class, methods, or variables defined as private can be accessed within the class only.
The below simple Java code explains the different access specifiers and how they work.
package myaccessdemoapplication;
// Default access (package-private)
// used when no access modifier is specified
class DefaultAccessClass {
int defaultVar = 10; // Package-private variable
void printDefaultMessage() {
System.out.println("Default method");
}
}
// Public access class
// Visible to all classes within and outside package
public class PublicAccessClass {
// Public variable
public int publicVar = 20;
public void printPublicMessage() {
System.out.println("Public method");
}
}
// Class for demonstrating
// private and protected access
class ProtectedAccessClass {
// Private variable
private int privateVar = 30;
protected void printProtectedMessage() {
System.out.println("Protected method");
}
}
// Subclass of ProtectedAccessClass
// to demonstrate protected access
class Subclass extends ProtectedAccessClass {
void accessPrintProtectedMessage() {
// Protected method
// can be accessed in the subclass
printProtectedMessage();
}
}
class Main {
public static void main(String[] args) {
// Accessing the DefaultAccessClass
DefaultAccessClass defaultObj = new DefaultAccessClass();
System.out.println("Default Variable: " + defaultObj.defaultVar);
defaultObj.printDefaultMessage();
// Accessing the PublicAccessClass
PublicAccessClass publicObj = new PublicAccessClass();
System.out.println("Public Variable: " + publicObj.publicVar);
publicObj.printPublicMessage();
// Accessing the ProtectedAccessClass class
ProtectedAccessClass accessDemoObj = new ProtectedAccessClass();
// Error: privateVar has private access
// System.out.println(accessDemoObj.privateVar);
// Error: printProtectedMessage has protected access
// accessDemoObj.printProtectedMessage();
// Accessing the Subclass
Subclass subclassObj = new Subclass();
// Error: privateVar has private access
// System.out.println(subclassObj.privateVar);
// Accessing printProtectedMessage through the subclass
subclassObj.accessPrintProtectedMessage();
}
}
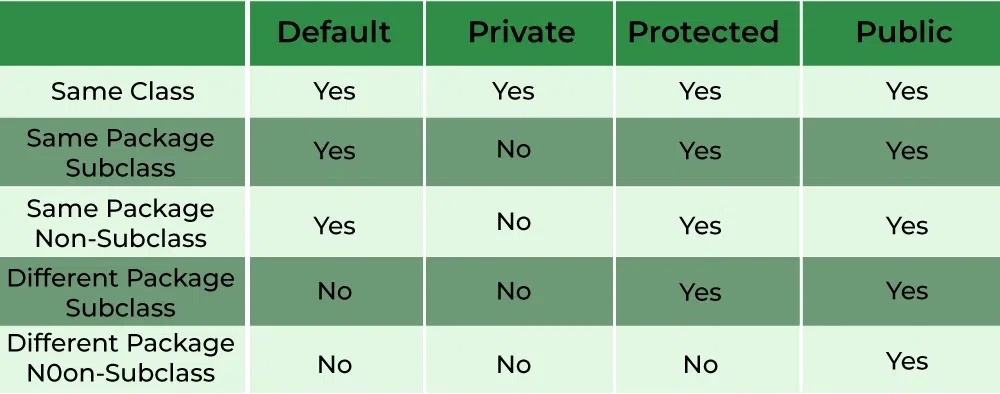