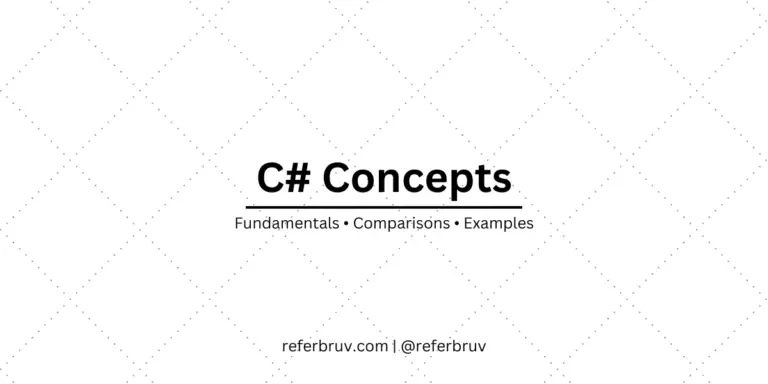
Easy Differences between Local Variable and Class Variable
Let us find out the top 5 differences between class variables and local variables based on their functionality and usage and summarize with examples.
Let us find out the top 5 differences between class variables and local variables based on their functionality and usage and summarize with examples.
In this article, let's explore the different keywords present in C# to implement method overriding with illustrating examples.
In this detailed article, let's understand what is Polymorphism, types, features and how it works with illustrating examples in C#
In this detailed article, let's explore the concept of Inheritance in Object Oriented Programming with illustrating examples
In this detailed article, let's explore what is abstraction in OOP with an illustrated example and understand how it works
In this article, let's understand the basics of encapsulation and why this is an important characteristic of any Object Oriented Language
In this article, let's look at how Garbage Collection works and things we need to keep in mind while developing memory optimized applications in dotne..
In this article, let's look at the two main concepts in LINQ execution patterns that help in writing efficient and memory conscious expressions.
class MyClass2
{
private static int _counter = 0;
public MyClass2()
{
if (_counter > 2)
{
throw new Exception("Instances created beyond limit");
}
else
{
++_counter;
}
}
public void ShowCounter()
{
Console.WriteLine($"Counter is at {_counter}");
}
}
MyClass2 c;
for (int i = 0; i < 5; i++)
{
c = new MyClass2();
c.ShowCounter();
}
output:
Counter is at 1
Counter is at 2
Counter is at 3
Unhandled exception. System.Exception: Instances created beyond limit