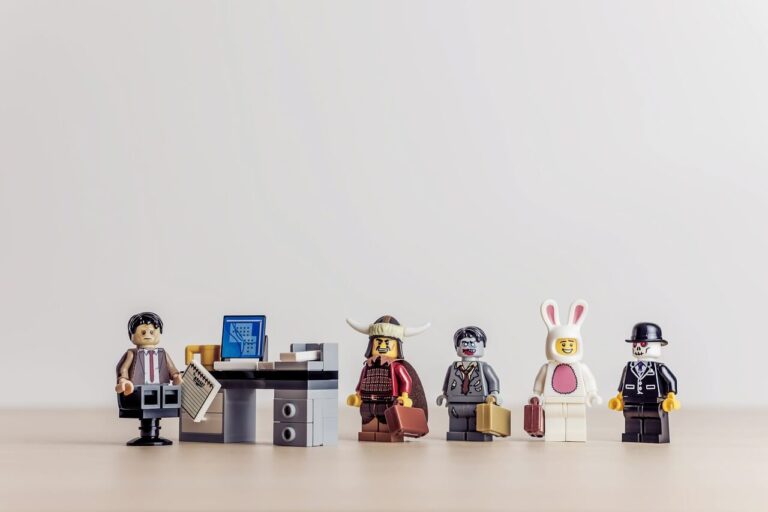
parseInt() And Number() In Javascript Made Easy
In JavaScript, you can use parseInt() or number() methods to convert string to a number. But There is a slight difference between how these methods work.
In JavaScript, you can use parseInt() or number() methods to convert string to a number. But There is a slight difference between how these methods work.
DOM API provides different methods to access elements in a document, via JavaScript. The following are the methods available.
Map, Reduce and Filter are three functions which operate on a given array of values.
let arr = [1, 2, 3, 4, 5];
let result2 = arr.map(function (current, index) {
return current % 2;
});
// output:
[ 1, 0, 1, 0, 1 ]
let result1 = arr.reduce(function (prev, current) {
console.log(`${prev}, ${current}`);
return prev + current;
});
// output:
1, 2
3, 3
6, 4
10, 5
result1: 15
let result3 = arr.filter(function (current, index) {
return current % 2 === 0;
});
// output:
[ 2, 4 ]
We can do it in three steps.
<!DOCTYPE html>
<html>
<body id="myBody">
<div id="mainContainer" class="container-fluid">
<div id="firstRow" class="row">
<div id="fullCol" class="col-md-12">
<h1 id="headingText">Hello World!</h1>
<p id="subHeadingText">
This is some sample text you're viewing on the Page.</p>
</div>
</div>
</div>
<script type="text/javascript">
(function () {
let elements = document.getElementsByTagName("*");
let ids = [];
for (let i = 0; i < elements.length; i++) {
let element = elements[i];
if (element.id) {
ids.push(element.id);
}
}
console.log(JSON.stringify(ids));
})();
</script>
</body>
</html>
output:
["myBody","mainContainer","firstRow","fullCol","headingText","subHeadingText"]
(function () {
let k = 1;
function kd() {
console.log(`value of k is ${k}`);
}
kd();
})();
output:
value of k is 1
function outer() {
let counter = 0;
function inner() {
counter++;
return counter;
}
return inner;
}
let instance = outer();
console.log(instance());
console.log(instance());
console.log(instance());
output:
1
2
3
console.log(`The value of x before is ${x}`);
var x = 10;
console.log(`The value of x after is ${x}`);
output:
The value of x before is undefined
The value of x after is 10
console.log(`The value of x before is ${x}`);
let x = 10;
console.log(`The value of x after is ${x}`);
output:
console.log(`The value of x before is ${x}`);
^
ReferenceError: Cannot access 'x' before initialization
In JavaScript, double equal operator (==) stands for value compare. The operands on either sides are converted into a common type and are compared for their values.
// JS converts the right type to equivalent left type
// 5 == '5'
// 5 == number('5') == 5
// result is true
5 == '5'
the triple operator (===) stands for both value and type compare. The operands are not type converted and instead are looked for their type match as well.
5 === '5' // result is false since we're comparing a number and a string
In JavaScript, we can get the browser information by means of the window.navigator object.
#properties#
window.navigator.appName
window.navigator.appCodeName
window.navigator.platform