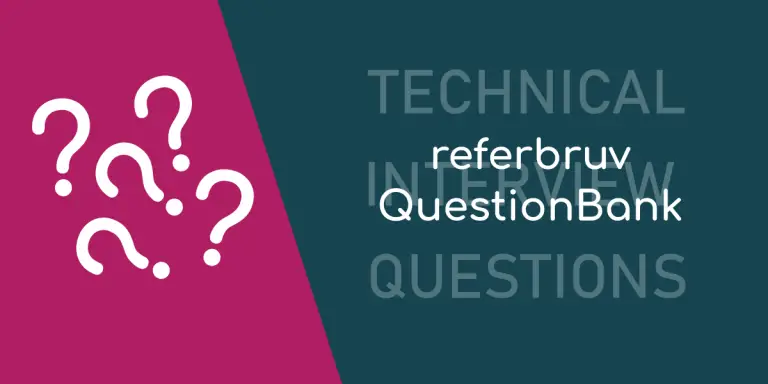
What are the different lifecycle hooks available to Components in Angular?
The below are the lifecycle hooks available in Angular. These are added by implementing the respective interface on the component.
- ngOnChange - before OnInit and whenever Input property changes in value
- ngOnInit - called once the Input properties are set, used to fetch initial data
- ngDoCheck - detect and act upon any changes that are by default on detected
- ngAfterContentInit - after loading any external entity into the component, such as a directive
- ngAfterContentChecked - after the content loaded is checked
- ngAfterViewInit - after the view is initialized
- ngAfterViewChecked - after the view is checked
- ngOnDestroy - just before the component is destroyed