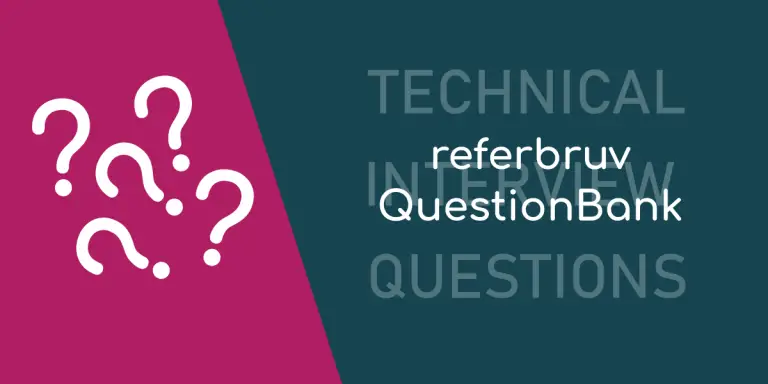
What are Delegates? How do you use them?
- A Delegate is a type which can help passing a function as a parameter - similar to pointer functions.
- These are derived from the Delegate class in .NET and are sealed types.
- The type of the delegate is determined by the name of the delegate.
- Methods passed into the delegate type must have the same signature as the defined delegate type.
- There are three steps:
- Declare a delegate type
- Instigate the type by associating with a method definition
- Invoke it
- Since the instantiated delegate is an object, it can be passed as a parameter, or assigned to a property. This allows a method to accept a delegate as a parameter, and call the delegate at some later time. This is known as an asynchronous callback, and is a common method of notifying a caller when a long process has completed.
void StartingPoint()
{
CallDelegate("HipHop", d1);
CallDelegate("HipHop", d2);
}
// call the passed Del reference
// with the passed parameter
void CallDelegate(string str, Del d)
{
// Step 3: Calling the delegate
d(str);
}
// Step1: declare a delegate type Del
delegate void Del(string str);
// Step 2: Instigation
Del d1 = delegate (string str)
{
Console.WriteLine($"Hello {str}");
};
// Step 2: Instigation
Del d2 = str =>
{
Console.WriteLine($"Hello there! {str} via a Lambda Expression.");
};
Output:
Hello HipHop
Hello there! HipHop via a Lambda Expression.