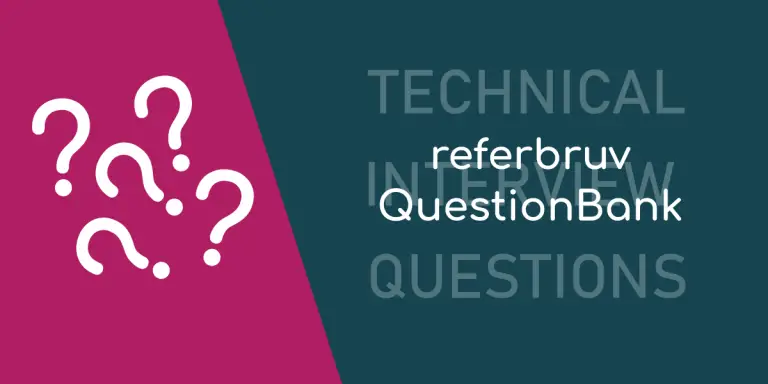
How can two components communicate with each other?
In Angular, components can communicate with each other by any of the following means:
- Input parameters
- Output parameters
- Service methods
- Subject and Observables
In Angular, components can communicate with each other by any of the following means:
To enable a class to be used under a using() block, the class must implement the IDisposable interface with its own implementation of the Dispose() method.
public class MyClass : IDisposable {
public void Dispose() {
// implementation of Dispose method
}
}
// in some other method
using(var myclass = new MyClass()) {
// myclass instance is alive inside
// this block only
}
Dispose is never called by the .NET Framework; you must call it manually - preferably by wrapping its creation in a using() block.
We can make use of the built-in UseExceptionHandler() middleware for catching Global Errors in ASP.NET Core.
app.UseExceptionHandler(err =>
{
err.Run(async ctx =>
{
context.Response.StatusCode = 500;
context.Response.ContentType = "application/json";
// gets error detail
var ex = context.Features.Get<IExceptionHandlerPathFeature>();
var error = new MyResponseModel() { Content = ex.Error.Message };
await context.Response.WriteAsync(JsonConvert.SerializeObject(error));
});
});
We can use raiseError to throw custom exceptions in an P-SQL block.
Example:
RAISERROR (N'Error Raised: %s %d.', -- Message text.
10, -- Severity,
1, -- State,
N'number', -- First argument from the
5); -- Second argument.
Returns Error Message: Error Raised: number 5.
To create a strongly-typed class for binding to a configuration section:
A Configuration section can be bound to a strictly-typed class object in two ways:
IOptionsMonitor is a part of the IOptions provided in ASP.NET Core. It returns the "CurrentValue" and notifications when options change.
Named options are when a single class is to be used for creating different objects of configurations that have the same structure.
The below are the differences between IOptions and IOptionsSnapshot explained respectively. Both are provided as part of the IOptions framework in ASP.NET Core