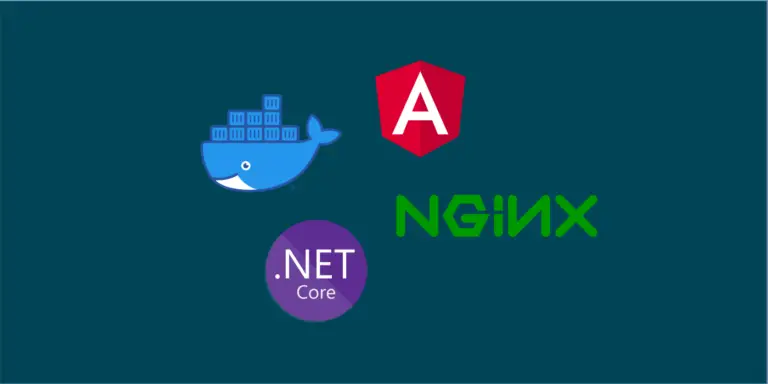
How to deploy full ASP.NET Core app in Docker
n this article, let's build a multi-container full stack application deployed through Docker Compose. We'll develop an Angular application that consum
n this article, let's build a multi-container full stack application deployed through Docker Compose. We'll develop an Angular application that consum
In this article, let's talk about how we can use docker in building and running an Angular application in a container with example.
We can do it in three steps.
<!DOCTYPE html>
<html>
<body id="myBody">
<div id="mainContainer" class="container-fluid">
<div id="firstRow" class="row">
<div id="fullCol" class="col-md-12">
<h1 id="headingText">Hello World!</h1>
<p id="subHeadingText">
This is some sample text you're viewing on the Page.</p>
</div>
</div>
</div>
<script type="text/javascript">
(function () {
let elements = document.getElementsByTagName("*");
let ids = [];
for (let i = 0; i < elements.length; i++) {
let element = elements[i];
if (element.id) {
ids.push(element.id);
}
}
console.log(JSON.stringify(ids));
})();
</script>
</body>
</html>
output:
["myBody","mainContainer","firstRow","fullCol","headingText","subHeadingText"]
(function () {
let k = 1;
function kd() {
console.log(`value of k is ${k}`);
}
kd();
})();
output:
value of k is 1
function outer() {
let counter = 0;
function inner() {
counter++;
return counter;
}
return inner;
}
let instance = outer();
console.log(instance());
console.log(instance());
console.log(instance());
output:
1
2
3
console.log(`The value of x before is ${x}`);
var x = 10;
console.log(`The value of x after is ${x}`);
output:
The value of x before is undefined
The value of x after is 10
console.log(`The value of x before is ${x}`);
let x = 10;
console.log(`The value of x after is ${x}`);
output:
console.log(`The value of x before is ${x}`);
^
ReferenceError: Cannot access 'x' before initialization
The below are the lifecycle hooks available in Angular. These are added by implementing the respective interface on the component.
To work with Cookies, we can make use of the CookieService which is a part of the ngx-cookie-service module.
npm install --save ngx-cookie-service
import { CookieService } from 'ngx-cookie-service';
@NgModule({
providers: [ CookieService ],
})
export class AppModule { }
constructor( private cookieService: CookieService ) {
this.cookieService.set( 'MyCookieKey', 'Hello There!' );
this.cookieValue = this.cookieService.get('Test');
}
In JavaScript, double equal operator (==) stands for value compare. The operands on either sides are converted into a common type and are compared for their values.
// JS converts the right type to equivalent left type
// 5 == '5'
// 5 == number('5') == 5
// result is true
5 == '5'
the triple operator (===) stands for both value and type compare. The operands are not type converted and instead are looked for their type match as well.
5 === '5' // result is false since we're comparing a number and a string