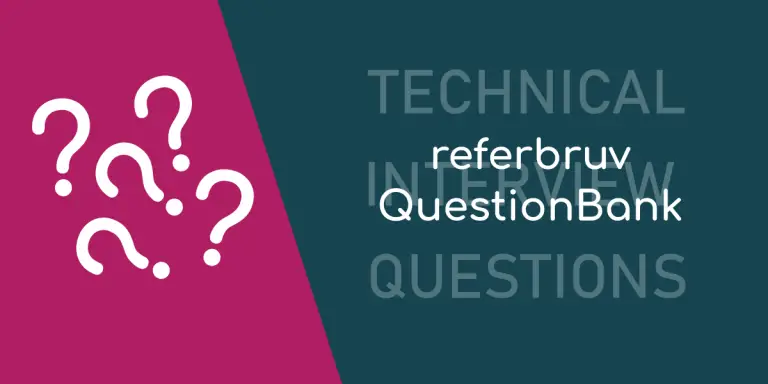
How to handle unmanaged code memory?
Unmanaged code refers to the components which are not a part of the CLR and which cannot be maintained by the Garbage Collector.
Unmanaged code refers to the components which are not a part of the CLR and which cannot be maintained by the Garbage Collector.
The answer is No. We cannot unit test a private method.
public class MyClass {
///
}
public static MyClassExtensions {
public static MyClass DoExtended(this MyClass obj, int someparam1, int someparam2.. )
{
// do something
return obj;
}
}
MyClass obj = new MyClass();
obj.DoExtended(); // this works although DoExtended is not a part of MyClass
IDisposable is an interface which provides a mechanism for releasing unmanaged resources which are not collected by Garbage Collector.
Garbage Collector is an important component, responsible for cleaning of memory when the resources are no longer required by the program.
(function () {
let k = 1;
function kd() {
console.log(`value of k is ${k}`);
}
kd();
})();
output:
value of k is 1
function outer() {
let counter = 0;
function inner() {
counter++;
return counter;
}
return inner;
}
let instance = outer();
console.log(instance());
console.log(instance());
console.log(instance());
output:
1
2
3
console.log(`The value of x before is ${x}`);
var x = 10;
console.log(`The value of x after is ${x}`);
output:
The value of x before is undefined
The value of x after is 10
console.log(`The value of x before is ${x}`);
let x = 10;
console.log(`The value of x after is ${x}`);
output:
console.log(`The value of x before is ${x}`);
^
ReferenceError: Cannot access 'x' before initialization
delegate void Del(string str);
// declaring an anonymous method
// assigning to a Delegate type Del
Del d1 = delegate (string str)
{
Console.WriteLine($"Hello {str}");
};
// declaring a lambda expression
// assigning to a Delegate type Del
Del d2 = str =>
{
Console.WriteLine($"Hello there! {str} via a Lambda Expression.");
};
Func<int, int> Sum = delegate (int a, int b) {
return a + b;
}
Action<int, int> PrintSum = delegate (int a, int b) {
Console.WriteLine(a+b);
}
// prints 9
Console.WriteLine(Sum(5,4));
PrintSum(5,4);